Have you ever been bored with developing iOS applications using MVC? Then, it is the right time to move on to the MVVM design pattern.
MVVM (Model View ViewModel) is a design pattern, which has been widely used as more event-driven apps have started emerging. A few years back, if anyone built a product, it has probably been built using MVC (Model View Controller). In recent times, the MVC architecture has lost its place as the default design pattern. There are several advantages of migrating to MVVM from MVC in case of iOS app development. You can also evaluate the available design patterns for iOS development to understand why to use MVVM beyond the MVC design pattern.
Understanding MVVM
Before we move further, you should understand two vital, integral parts of the iOS applications: UI logic & Business logic.
- UI logic is the part of the program that handles the user interface like what the user perceives & interacts with. It includes updating the table, the labels and animating the screen components.
- Business logic is the underlying process that handles communication between databases and the end-user interface. It guarantees the model is updated, the data is handled in a way designed and so on. It includes actions like deleting items in a list, updating the data, and formatting a data type.
MVVM is derived from the MVC pattern and addresses the issues of previous MV (X) patterns. Here is the architecture of MVVM:
In the MVVM design pattern, view and controller are closely coupled together and treated as one component. It can also be represented as UIView or UIViewController objects, accompanied by their .xib and .storyboard files.
Previously, the ViewController was overloaded with activities like networking, handling of signals from UI, collecting back the response, etc. MVVM introduced a new component called ViewModel, which divided and delegated the responsibility of the ViewController.
Components
- Model – Acts the same as in MVC design patterns – it is used by ViewModel and updates whenever ViewModel sends a new update.
- ViewController – It is responsible for setting up UI views. There is no direct interaction between the ViewController & Model and it no longer needs to worry about business logic. It should go through ViewModel and request for what it requires in a ready-to-display format.
- ViewModel – It is responsible for all business logic. If the ViewController has a label, it triggers entire calls and sends & receives data. It is completely independent of the ViewController. It receives information from the ViewController, processes all this information & sends the results back to ViewController.
The ultimate goal of MVVM is to isolate the UI logic responsibility of ViewController to ViewModel. In addition, ViewModel hides data preparation code for visual presentation, asynchronous networking code as well as code listening for model updates. Further, when the ViewModel needs to communicate anything to the View, it will accomplish this with data bindings.
MVVM Swift Example to create a Login Screen
In this example, we will create a login function that handles data inside the ViewModel. The iOS development in Clarion consistently involves in feature-rich, interactive & secure iOS app. To keep this example simple, we will cover only the essential aspects. We ignored the autolayout portion as well as interface builder changes & focused only on the connection between ViewController and ViewModel.
ViewController
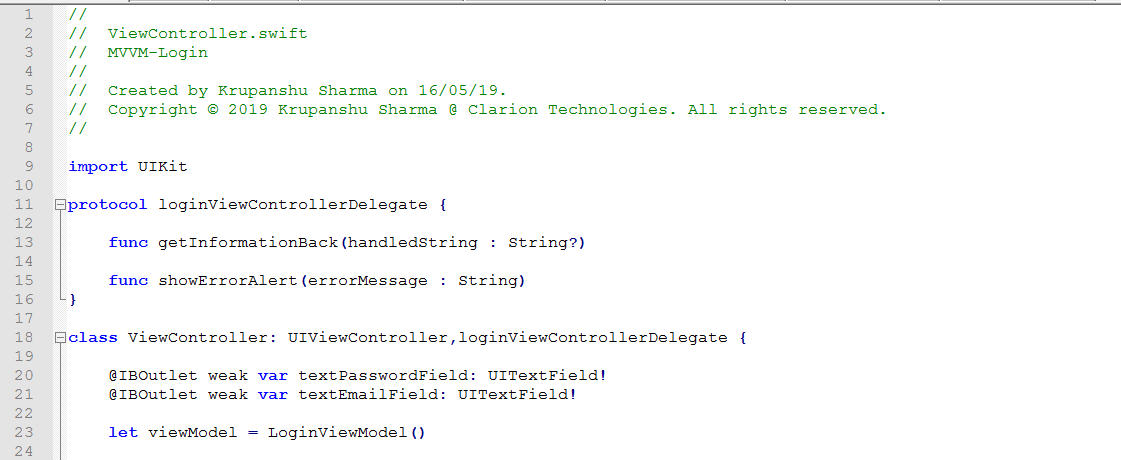
The biggest enhancement of MVVM is to move the fetching as well as caching logic from the ViewController to the ViewModel. The responsibility of ViewController is to only to show up and get details from the UI. We applied these points in the above code:
- The code gets the data from UI – the value from the text box
- Respond to the data from ViewModel – Shows the result based on the signal from ViewModel
- Involves protocol called ViewControllerDelegate – Ensures that the ViewController does not need to care about what the ViewModel did, just display the final output of the operation.
- Includes getInformationBack – we can get values from ViewModel through this method.
ViewModel
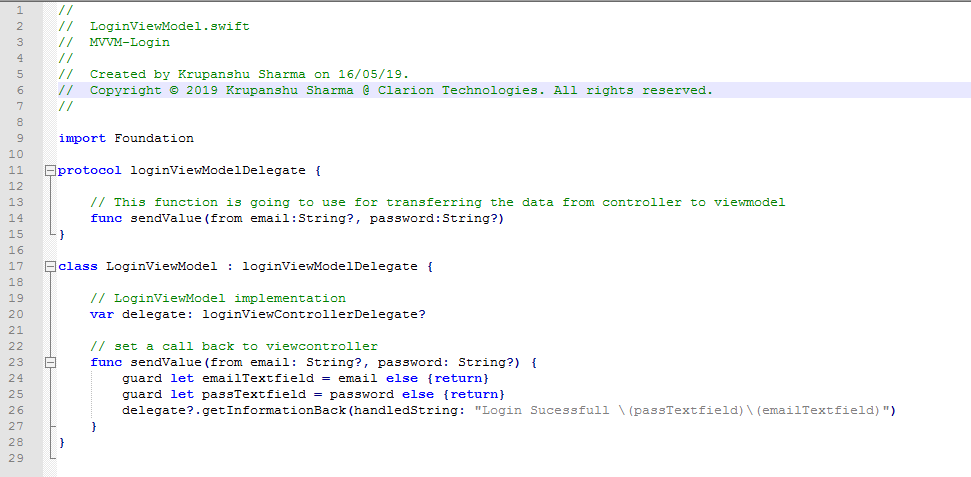
We can use the string extension for validation like below:
Validation Extension
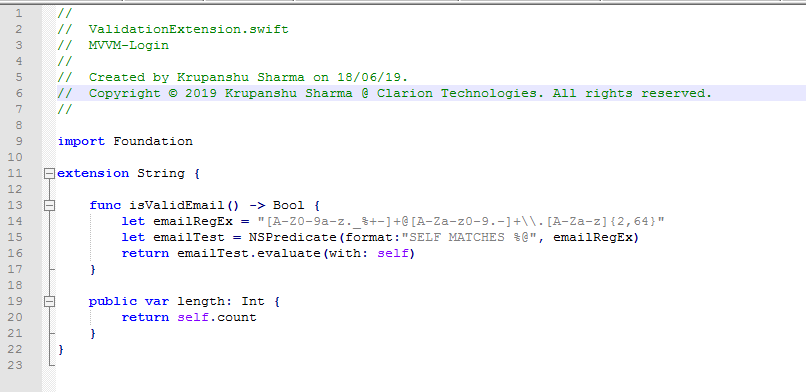
Similarly, ViewModel does not need to care about where and how ViewController obtained the data. Its sole concern is what to do with the data. In the above ViewModel code, ViewModel gets an email and password text from the ViewController and checks for validation and passes the information back for further processing.
As such, all the responsibilities are isolated, ensuring every component is independent of each other.
Here is an execution of the code with the output shown below:
With MVVM, now you have extracted the responsibilities like data gathering/transforming/logic from the ViewController.
Benefits of MVVM
Beyond the benefit of separating the responsibilities among the components, MVVM includes other benefits too, and they are as follows:
- Enhanced Testability – View and Controllers are notoriously difficult to test thanks to its association to the view layer. By moving data manipulation to the ViewModel, MVVM makes the testing much easier. Unit testing of ViewModel is easy now, as it doesn’t have any reference to the object it’s maintained by. Similarly, testing of ViewController also becomes easy since it no longer depends on the Model.
- Transparent Communication - The ViewModel offers a transparent interface to the ViewController that it uses to interact with the model and view layers. This results in a transparent communication between the layers of the applications.
MVVM is an excellent design pattern, which reduces the amount of code on the Views and keeps the code clean, reusable, maintainable and testable, no matter what programming language we are employing. Our mobile application developers love it since it enables us to offer our clients a high-quality code. On understanding these features, almost all iOS development companies encourage you to go with this platform for iOS development.