Decoding Java Microservices: Are You Ready for the Next Evolution in Software Architecture?
Microservices in Java are an architectural approach in software development and involve using various small modules and components to achieve specific business goals. These modules have APIs that communicate with each other, and microservices follow a service-oriented approach.
Java applications that use microservices perform better than others and are compatible with all languages, meaning there are no performance or resource usage barriers. Developers can enjoy enhanced flexibility and build customized applications with Java microservices, and it is one of the hottest trends in software development. Even top companies like Amazon, Netflix, Uber, Spotify, and SoundCloud leverage the Java microservices architecture, and it pairs well with different programming languages like Java, Node.Js, PHP, etc.
Below, we cover everything you need about the Java microservices architecture.
Monolithic vs. Microservices Architecture
A monolithic architecture uses a single unit as the base for all functional components. All components are run using a single codebase where all updates are made, and scaling applications become tricky since several components exist. The more features added to applications, the larger the codebase becomes, thus limiting flexibility and space. Monolithic architecture covers background processing, business logic, database operations, and other organizational functions deployed simultaneously. A single error can cause the entire application to crash and lead to operational failures or delays.
Java microservices architecture follows a different approach where business services are represented as separate services instead of functioning as a single unit. Java microservices architecture uses different codebases for API communications, and each function can be scaled independently. If one service goes down, it doesn't affect the entire application, and the other components continue functioning as intended.
Characteristics of Java Microservices
The following are the main characteristics of Java microservices:
#1. Fail-Proof
The best benefit of using a Java microservice architecture is that it is essentially fail-proof. Microservices applications can divert traffic to several components and are very reliable. Organizations can hire Java developers to find bugs and avoid clashes with other components.
#2. Easy Routing
Java microservices transmit data through wires to multiple components. Wires process requests and provide the necessary outputs for consecutive components. It provides the best possible routing, receives requests, and uses business logic to deliver the best responses.
#3. Multiple Components
Projects designed with the Java microservice architecture can be divided into multiple components. Each developer can focus on specific tasks, and several components are deployed, tested, and updated at different rates. It makes it easy to collaborate on multiple projects as well.
How the Microservices Architecture Works
The Java microservices architecture consists of several components, namely:
- API Gateway – The API gateway forwards calls to specific services on the backend and can collect responses from different services. It also returns responses to clients.
- Microservices – Java microservices help divide existing services into more minor services to carry out different business capabilities such as managing orders, wishlists, user registrations, etc.
- Database – Microservices use an independent database or a shared database
- Inter-microservices communication involves using the REST or Messaging as the key protocol for interaction and communication.
How to Implement Java Microservices Architecture?
Implementing a microservices architecture in Java involves breaking down a monolithic application into smaller, independently deployable services that communicate with each other. Implementing Java microservices specifically with a Java framework involves a strategic selection of tools and practices tailored to the application's needs. Below are the general steps you can follow to implement Java microservices architecture:
1. Define Microservices Boundaries:
Identify and define the boundaries of your microservices. Each microservice should have a specific and independent business functionality.
2. Choose a Microservices Framework:
Select a Java microservices framework that facilitates the development and deployment of microservices. Popular choices include Spring Boot, Quarkus, and Micronaut.
3. Set Up Development Environment:
Install the necessary development tools, including the Java Development Kit (JDK), Integrated Development Environment (IDE), and the chosen microservices framework. Popular IDEs for Java development include IntelliJ IDEA and Eclipse.
4. Service Discovery and Registration:
Implement a service discovery mechanism to allow microservices to find and communicate with each other. Tools like Eureka (from the Spring Cloud stack) or Consul can be used for service discovery.
5. API Gateway:
Create an API Gateway to manage and route requests to the appropriate microservices. Tools like Spring Cloud Gateway or Netflix Zuul can be used for this purpose.
6. Communication Protocols:
Decide on communication protocols between microservices. RESTful APIs and message queues (e.g., Apache Kafka or RabbitMQ) are common choices.
7. Data Management:
Choose how data will be managed across microservices. Each microservice should have its own database, and data consistency can be maintained through techniques like event sourcing or distributed transactions.
8. Implement Security Measures:
Implement security measures such as authentication and authorization. Use tools like OAuth, JWT (JSON Web Tokens), and secure communication protocols (HTTPS) to ensure the security of microservices interactions.
9. Monitoring and Logging:
Implement monitoring and logging to track the performance and health of your microservices. Use tools like Prometheus, Grafana, and ELK stack (Elasticsearch, Logstash, Kibana) for centralized logging and monitoring.
10. Testing:
Adopt a comprehensive testing strategy. Unit tests, integration tests, and end-to-end tests are crucial to ensure the reliability and stability of each microservice.
11. Containerization and Orchestration:
Containerize your microservices using technologies like Docker. Adopt container orchestration tools like Kubernetes to manage the deployment, scaling, and monitoring of your microservices.
12. Continuous Integration/Continuous Deployment (CI/CD):
Implement CI/CD pipelines to automate the building, testing, and deployment of microservices. Tools like Jenkins, GitLab CI, or GitHub Actions can be used.
13. Fault Tolerance and Resilience:
Design your microservices with fault tolerance in mind. Implement retry mechanisms, circuit breakers, and fallback strategies to handle failures gracefully.
14. Documentation:
Document each microservice's API, dependencies, and usage. This documentation is crucial for developers who need to integrate with or maintain the microservices.
15. Scalability:
Design your microservices to be scalable. Each microservice should be able to scale independently based on the demand for its specific functionality.
16. Deployment:
Deploy your microservices to a production environment. Make use of container orchestration tools for managing deployments and ensuring high availability.
17. Monitoring and Optimization:
Continuously monitor the performance of your microservices in a production environment. Optimize and scale as needed based on usage patterns and resource requirements. Our company is among the top Java development companies leading the way in the software development scene.
Why Use Java Microservices Architecture?
Here is why you may want to consider using the Java microservices architecture for your web development projects:
#1. Multiple Frameworks
Java microservices can work with several frameworks and make it easier and faster to design the latest projects. Many services like Spark, Boot, and Jersey are compatible with the Java microservices architecture. These frameworks provide simple configurations and help developers establish communication between microservices. Many features are available in Java that can be used to develop custom microservices.
#2. Great Community Support
One of the many reasons Java microservices are becoming so popular these days is because of excellent community support. The programming language has multiple libraries and can develop complex apps and websites by being dependent on the Java Virtual Machine (JVM). You can hire Java developers to build enterprise-grade apps, cloud-based applications, big data projects, and for backend development. Java microservices seamlessly integrate with AI and advanced technologies, making it future-proof.
#3. Simplified Syntax
Java syntax is easy for new developers to pick up, and there isn't a huge learning curve. Naturally, Java microservices support the latest standards of Java EE for data handling, CDIs, JAX-Rs, and many more. The Java development process enables developers to create microservices and implement them into projects without facing issues such as overheads.
Advantages of Java Microservices
There are several reasons why organizations should use Java microservices:
#1. Productivity
Java microservices architecture is perfect for large teams and best suited for completing large-scale projects. The microservices approach allows organizations to split up into multiple components and work on the same project without any coordination. Teams can work independently and have the freedom to select the language they want to write a microservice in and use different tech stacks to achieve different objectives.
#2. Agility & Reusability
Being Agile is one of the top priorities of today's organizations, and Java microservices architecture grants project autonomy. Developers can make more efficient deliveries and enjoy shorter development cycles. Microservices represent different features of applications that function independently and can make projects less complex. Developing the entire application becomes effortless, ensuring overall safety and success.
Changes can always be made to the features; if a particular feature doesn’t work or becomes outdated, it can be easily replaced. Many of these features are also reusable and can serve as a base to add new features.
#3. Real-Time Processing
Continuous testing and deployment are significant parts of the software application development lifecycle. Java microservices help process products in real-time and can immediately find bugs to address them. It can perform instant revisions and renewals, assisting developers with the real-time processing function.
#4. Portability
Developers can cut down on maintenance costs, optimize development, and improve resource allocation. Companies can launch and move services independently, easily transition between platforms, and utilize various infrastructure options effectively. There are many affordable deployment and migration plans coupled with these services.
Use Cases of Java Microservices
Businesses are moving away from legacy platforms and embracing Java microservices to modernize their IT infrastructure. Various applications have been built and deployed using a microservices architecture in recent years.
The following are the most common use cases of Java microservices:
#1. Modernize and Refactor Legacy Applications
Java microservices help applications apply constant updates and adapt to changing market demands and trends. It adds extra features and functionalities without impairing existing ones.
#2. Extensive Data Management
Java microservices architecture is an excellent choice for developing complex apps for multi-cloud environments. It can classify data and separate it into different components for easier management.
#3. Multi-Group Application Development
Java microservices allow multiple developers to work on the same project and make changes to the application. It expedites the development process and prevents potential issues that may crop up from a monolithic approach in the coming years. Independent components in these services can be separated or combined into different groups to match project requirements.
#4. Big Data Applications
To build complex applications, Java microservices can be combined with event-driven architecture (EDA). A classic example is the case of Amazon, which used Java microservices to simplify its application deployment pipeline and identify potential problem areas. eBay managed its growing traffic volumes and database calls by leveraging the Java microservices architecture, and it helped keep the company’s website stable, thus boosting time-to-market rates.
#5. Machine Learning
Another great use case of Java microservices architecture is Machine Learning (ML). Microservices-based ML environments can analyze dataflows and make accurate predictions. Several great M frameworks like Apache Mahout, Apache Singa, and TensorFlow are compatible with the Java microservices architecture, and multiple machine learning frameworks can be included with a single workflow to create multiple models on the same data flow. Developers can run multiple frameworks simultaneously to get more outcomes and compare models to determine the best results.
#6. DevOps Workflows
Microservices and DevOps can work together to boost the productivity of DevOps teams in organizations. Java microservices provide faster dependencies and can enhance business operations by fixing project build configurations and scripts. Modern companies use Java microservices to build applications across multiple channels, such as hotel booking services, notification systems, login portals, etc. Every company can use it to foster innovation, thus enhancing business efficiency, scalability, and growth.
Read our new blog for insights on developing mobile applications with Java.
Conclusion:
Now that you know how Java microservices can streamline software application development and provide flexibility for building complex projects, you can use this technology well. Java microservices architecture helps developers add flexibility and makes applications more resilient and scalable. You can hire Java developers and start building your web application today using the Java microservices architecture to adopt its benefits.
Suppose you are considering using Java development services for microservices but aren’t sure how to get started. In that case, you can contact us or try our DevOps consulting services to receive insights.
Author
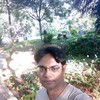