Deep dive into Java development and explore best practices that developers should implement.
Java Best Practices to Enhance Development Process
Java best practices include many strategies and techniques for effective, efficient, and maintainable code. Let's delve into some best practices while developing any Java project. Our experts have shared their opinions as we are among the top Java app development companies to help you enhance your development process and projects.
#1. Use Immutable Objects:
Creating immutable objects means that once an instance of the class is created, it cannot be changed. This approach is safer because it prevents the program from changing values in ways that could cause errors or require constant management. If making an immutable class is impossible, limit how much and which parts can be changed. Limiting mutability can reduce the risk of bugs and make the overall program easier to understand.
Use case example for clients: In a financial service application, Java developers can create immutable objects for transaction details. This ensures that once a transaction is recorded, its details can't be altered, preventing any discrepancies due to accidental or unauthorized changes. This approach increases the security and reliability of transaction processing.
#2. Use of generics:
Generics in Java allow you to create classes, methods, and interfaces that operate with different types of data. This means you can write a single class or method that can handle various data types, like integers, String, or any user-defined data types, without the need to rewrite the code for each type.
Use case example for clients: For a healthcare data management system, generics were used to create a unified API that could handle different types of medical records (like prescriptions, patient laboratories, and lab results) without making a separate code base. This not only simplified the code but reduced bugs related to type errors.
#3. Reduce Variables:
Keep variables as limited as possible, its one of the best practices for Java clean coding maintainability and readability. Declare variables where they are needed and not before, often just before their first use.
Use case example for clients: In a real-time trading platform, limiting the scope of variables improves the readability and code maintainability. Variables were declared within the scope of loops or conditions reducing the side-effects and making the code easier to debug.
#4. Concurrency utilities:
Instead of traditional thread management and synchronization techniques, leverage Java's concurrency utilities, like 'java.util.concurrent' package. Tools like 'ExecutorService', 'Future', and ConcurrentHashMap' help manage threads safely and efficiently.
Use case example for clients: In an ecommerce backend use Java's 'executor service' to handle multiple user checkout processes concurrently. With this approach, java developers can maximize the efficiency of server resources and reduce the response time during high traffic periods, enhancing user experience.
#5. Avoid Premature Optimization:
It can lead to complex and unreadable code. Focus on writing clear and simple code; optimize only after profiling the application to identify real bottlenecks.
Use case example for clients: Java developers can focus on achieving functional requirements before optimizing. A logistic software project used profiling tools post-deployment to identify and address performance bottlenecks, specifically in route calculation algorithms. It leads to significant performance improvements without complicating the initial development.
#6. Use Enuns Instead of Constants:
Java enums are very powerful, more than constants. They have fields, methods, and constructors. Using enums ensures type safety, and you can benefit from additional compile time checking.
Use case example for clients: A sport management application used enums to define player statuses (ACTIVE, INJURED, RETIRED). This allowed Java developers for safer updates and manipulations of player statuses across the system, leveraging type safety provided by enums.
#8. Functional Interfaces and Lambda Expressions:
Java 8 introduced lambda expressions and functional interfaces, which can significantly simplify your code. These features encourage a functional programming style, leading to more style concise and expressive code.
Use case example for clients: In a cloud storage solution, lambda expressions enhanced file filtering operations, allowing users to search for files based on multiple criteria (like date, size, type) more succinctly and flexibly.
#9. Avoid returning nulls:
Returning nulls from methods can be problematic, forcing callers to handle null conditions. Instead, consider using the Optional class introduced in Java 8 for methods that may not always return a value.
Use case example for clients: In a customer: In a CRM system, the use of 'Optional' in API responses prevented null pointer exceptions when data was unavailable, leading to more robust error handling and reducing crashes in consumer applications.
#10. Java Bean Conventions:
Adhering to JavaBean naming conventions (like using 'get', 'set', and 'is' prefixes appropriately) is not just about consistency; it also enables libraries and frameworks to easily interact with your classes, using reflection to manipulate properties programmatically.
Use case example for Clients: For a smart home system, adhering to JavaBean conventions allowed external frameworks to easily interact with device properties (like power status and device type), enabling dynamic property manipulation and simplifying the user interface logic.
#11. Resource management:
Ensure resources such as streams, connections, and files are closed after use. The try-with-resources statement introduced in Java 7 effectively handles this, guaranteeing that each resource is closed at the end of the state.
Use case example for clients: Java developers implemented video processing software to manage video streams. They ensured that all file handles were properly closed for processing, which prevented memory leaks and allowed the system to handle larger video files.
Our case study -
Discover how our Java developers implemented legacy migration for our clients!
What are the best practices for legacy modernization in Java?
Here are different types of Java best practices for legacy modernization:
1. Containerization:
Containerize legacy Java applications using technology like Docker and Kubernetes. This encapsulates the application in a controlled environment with all the necessary configurations and dependencies. Containerization not only optimizes resource use but also simplifies deployment and scaling processes, making applications more agile and responsive to the market needs.
2. Adopt microservices architecture:
Transition from monolithic architecture to microservices. This approach allows independent deployment of application components, which improves fault isolation and makes continuous deployment
3. Transition from monolithic architecture to microservices.
It allows independent deployment of application components, which improves fault isolation and makes continuous integration and continuous deployment (CI/CD) processes more effective. Use Spring Boot and Spring Cloud to facilitate this transformation, as these frameworks provide comprehensive support for developing microservices in Java.
4. Refactor for Cloud compatibility
Refactoring applications from cloud native allows them to be compatible with cloud scalability, resilience, and cost-effectiveness. This involves adopting stateless designs and integrating with cloud management services.
5. Implement Robust Security Practices:
Address security concerns inherent in legacy applications by integrating modern security practices. Use libraries that support automatic security updates and patches. Consider adopting OAuth for authentication and JWT for secure communication in your applications. Ensure that all dependencies are up to date with their secure versions to prevent vulnerabilities.
6. Enhance User Experience with Modern UI/UX Principles:
Modernize the user interface and user experience of your applications. Implementing responsive design and accessibility standards can significantly improve the performance of java applications, usability and accessibility of your applications. Consider frameworks like Angular or React to create interactive and modern web frontends.
7. Automate Testing and Deployment:
Utilize automation tools for testing and deploying applications to ensure high-quality releases and fast turnaround times. Integrate Frameworks like JUnit, Mociti, and Selenium for unit testing and UI testing. Employ Jenkins, GitLab CI, or GitHub Actions for automating builds, tests, and deployments.
Expert Tips to Help You Guide in Implementing Best Practices:
1. Understand the purpose and implications before implementing Java programming best practices.
2. Ensure that Java environment is up to date and that you are using the latest tools and frameworks that support best practices.
3. Make code quality a priority from the start.
4. Code reviews ensure all team members adhere to best practices
5. Automation tools like SonarQube, PMD, help detect coding flaws and adherence to coding standards.
6. Write unit test along your code.
7. Document and maintain a clear code, write comments, explain naming conventions, and a logical structure.
Conclusion:
Implementing these best practices of Java coding into your development process, you can enhance the quality, performance, and reliability of your Java applications. Hire Java programmers to develop more sustainable and easier applications and to implement java security best practices in the long run.
Author
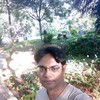