Explore how async/await in Python enhances scalability for high-performance applications.
In the evolving software development landscape, the need for high-performance I/O-bound applications has never been more critical. With Python's asynchronous programming capabilities, mainly through the async and await syntax, developers can harness the power of non-blocking I/O operations, enabling the construction of highly scalable and responsive applications. This blog post delves into the concepts of asynchronous programming in Python, its benefits, and practical examples to guide you through adopting async/await in your projects.
Looking to elevate your project with high-performance, scalable solutions? Hire Python developers today and transform your vision into reality with cutting-edge technology!
Understanding Asynchronous Programming
Asynchronous programming is a paradigm that facilitates concurrent execution of tasks without blocking the program's flow. Traditional synchronous I/O operations wait for the task to complete before moving to the next one, leading to inefficient use of resources. Asynchronous programming, on the other hand, allows tasks to run independently, improving throughput and responsiveness in I/O-bound applications such as web servers, database applications, and data processing systems.
The Evolution of Asynchronous Programming in Python
Python's journey towards asynchronous programming began with generators and the yield keyword, evolving through various iterations such as asyncio, a library introduced in Python 3.4, to the more intuitive, streamlined async/await syntax introduced in Python 3.12. This evolution signifies Python's commitment to providing a robust framework for writing asynchronous code that's both efficient and readable.
The Basics of async and await
The async and await keywords are the cornerstones of asynchronous programming in Python. Here's a quick overview:
- async: Used to declare a function as an asynchronous function. An async function is a function that is expected to perform an asynchronous operation.
- await: Used to pause the execution of the async function until a specific asynchronous operation is completed, allowing other tasks to run during the wait time.
Why Adopt async/await?
- Performance: By enabling non-blocking I/O operations, async/await allows for significant performance improvements in I/O-bound applications.
- Simplicity: The syntax is intuitive and easy to read, making asynchronous code look and behave more like traditional synchronous code.
- Efficiency: It facilitates efficient CPU and memory use, allowing for handling thousands of concurrent connections with minimal overhead.
Getting Started with async/await
Let's dive into how to implement async/await with a simple example:
python
Practical Applications
- Web Development: Frameworks like FastAPI and AIOHTTP leverage async/await to handle web requests asynchronously, improving throughput and scalability.
- Database Access: Asynchronous database libraries such as aiomysql and aiopg allow for non-blocking database operations.
- Data Processing: Asynchronous programming can significantly speed up data processing tasks, especially when dealing with I/O-bound operations like reading from or writing to a file system. Discover the leaders in innovation with our list of the Top 10 Python Development Companies in 2024. Elevate your project by partnering with the best in the business today!
Python's async/await syntax, introduced in Python 3.5, represents a significant advancement in writing asynchronous code, particularly for building high-performance I/O applications. This approach differs from traditional synchronous programming and earlier asynchronous solutions in Python in several key ways, offering a more efficient, readable, and scalable way to handle I/O-bound tasks. Let's delve into what makes async/await stand out:
Asynchronous vs. Synchronous Programming
- Non-blocking I/O Operations: Traditional synchronous operations wait for the task to complete before moving on to the next. In contrast, async/await allows for non-blocking I/O operations, where the system can initiate an I/O operation and execute other tasks without waiting for the operation to complete. This is particularly beneficial for high-performance web servers, database operations, and any application requiring concurrently handling numerous I/O operations.
- Improved Responsiveness: Applications using async/await can handle more tasks simultaneously by not blocking the main thread. This leads to significantly improved responsiveness and user experience in web services and data processing systems applications.
The graph above compares asynchronous and synchronous programming in terms of non-blocking I/O operations and improved responsiveness. As depicted, asynchronous programming significantly outperforms synchronous programming in both categories. This illustrates the efficiency and speed advantages of using asynchronous (async/await) techniques for handling multiple I/O operations concurrently and improving the responsiveness of applications, especially in web services and data processing systems.
Evolution of Asynchronous Programming in Python
- Generators and Coroutines: Before async/await, Python used generators and coroutines (with the yield and yield from syntax) for asynchronous programming. This approach was more complex and less intuitive, making the code harder to read and maintain.
- asyncio Module: Introduced in Python 3.4, the asyncio module provided a framework for writing single-threaded concurrent code using coroutines, event loops, and I/O scheduling. However, the syntax and patterns required to use asyncio effectively were still considered cumbersome by many python developers.
- async/await Syntax: With Python 3.5, the introduction of async and await keywords significantly simplified asynchronous programming. This new syntax made asynchronous code easier to write, read, and understand, bringing it closer in appearance to its synchronous counterpart.
Uncover the power of Python and its advantages over Java to streamline your development process. Learn why Python is the smart choice for modern applications today!
Key Features and Benefits
- Simplified Syntax: The async/await syntax is clear and straightforward, making asynchronous code more readable and maintainable.
- Efficient Concurrency: It enables efficient concurrency, allowing python developers to write code that performs multiple operations simultaneously, effectively utilizing system resources and reducing wait times associated with I/O operations.
- Error Handling: Asynchronous code using async/await can leverage traditional try-except blocks for error handling, making catching and managing exceptions easier.
- Integration with Asynchronous Libraries: There's a growing ecosystem of libraries designed to work with Python's asynchronous programming model, covering web frameworks, database connectors, and more, further extending the utility and power of async/await for high-performance applications.
Considerations
- Suitable for I/O-Bound, Not CPU-Bound Tasks: The primary advantage of async/await lies in I/O-bound applications. For CPU-bound tasks, using multi-threading or multi-processing might be more appropriate.
- Learning Curve: Despite its simplified syntax, there's a learning curve to understanding asynchronous programming concepts, such as event loops and coroutines.
- Debugging Complexity: Debugging asynchronous code can be more challenging due to its concurrent nature, requiring python developers to think differently about the flow of their programs.
Why Businesses Should Consider Python With Async/Await For Building Applications
Enhanced Performance through Non-blocking I/O
One of the most significant advantages of using Python's async/await is the ability to perform non-blocking I/O operations. Unlike traditional synchronous operations that require the execution to wait for a task to complete, async/await allows other tasks to run concurrently. This means a web server can handle more requests, a database application can execute more queries, and a data processing system can process more data in less time. For businesses, this translates to faster response times, improved user experiences, and the ability to handle high-traffic volumes or large datasets more efficiently.
Simplified Code for Complex Operations
The async/await syntax in Python enhances performance and simplifies the codebase for complex asynchronous operations. Traditional asynchronous programming can be challenging to write and maintain, often leading to a tangled web of callbacks. With async/await, the code looks and behaves more like traditional synchronous code, making it easier for python developers to read, write, and maintain. This simplicity reduces development time and costs, making it a cost-effective solution for businesses.
Scalability and Resource Efficiency
As businesses grow, their software needs to scale. Python's asynchronous programming model is inherently more scalable than its synchronous counterpart. It can handle thousands of concurrent connections with minimal overhead, making it an ideal choice for applications that need to scale up based on demand. Furthermore, async/await efficiently uses CPU and memory resources, allowing businesses to optimize their infrastructure costs while maintaining high performance.
Broad Ecosystem of Asynchronous Libraries
The Python ecosystem is rich with libraries and frameworks that support asynchronous programming. This includes web frameworks like FastAPI and AIOHTTP, database connectors such as aiomysql and aiopg, and many others designed for asynchronous operations. This ecosystem allows businesses to leverage existing solutions for everyday tasks, speeding up development and ensuring that applications are built on proven reliable components.
Forward-Looking Technology
Python's commitment to supporting asynchronous programming through the evolution of async/await syntax indicates its position as a forward-looking technology. By choosing Python for software development, businesses align themselves with a technology adaptable to future performance and scalability requirements. This foresight ensures that applications remain competitive and meet user expectations as technology evolves.
Considerations for Adoption
While the benefits are clear, businesses should consider the learning curve associated with asynchronous programming. Python developers may need time to become proficient in these concepts. However, the long-term benefits of improved performance, scalability, and efficiency outweigh the investment in learning and development.
Comparison Table Involving Evaluating Python's Async/Await Against Other Popular Frameworks
Creating a comparison table involves evaluating Python's async/await against other popular frameworks and languages that support asynchronous programming for high-performance I/O applications. Here, we'll compare Python with Node.js, Go (Golang), and Java (using the CompletableFuture API and reactive frameworks like Spring WebFlux). This comparison will focus on key aspects such as ease of use, performance, scalability, ecosystem, and community support.
Feature / Framework |
Python (async/await) |
Node.js |
Go (Golang) |
Java (CompletableFuture & Spring WebFlux) |
Ease of Use |
High |
Medium |
High |
Medium |
Performance |
High |
High |
Very High |
High |
Scalability |
High |
High |
Very High |
High |
Ecosystem |
Rich |
Very Rich |
Rich |
Very Rich |
Community Support |
Strong |
Very Strong |
Strong |
Strong |
Non-blocking I/O |
Native Support |
Native Support |
Native Support |
Native Support |
Concurrency Model |
Coroutine-based |
Event-loop |
Goroutines |
Thread-based & Reactive |
Syntax Simplicity |
Very Simple |
Simple |
Simple |
Complex |
Error Handling |
Traditional Try/Catch |
Callbacks & Promises |
Traditional Try/Catch |
Future-based & Reactive |
Ideal Use Case |
Web servers, Asynchronous data processing |
Real-time applications, Single-page applications |
Concurrent services, Networked applications |
Web applications, Microservices |
- Ease of Use: Python's async/await syntax is very intuitive and simplifies asynchronous programming, making it more accessible to python developers familiar with synchronous coding patterns. Node.js uses callbacks and promises, which can lead to callback hell if not properly managed, though modern async/await syntax in ES7 improves this. Go's simplicity and powerful standard library make concurrency models easy to implement. Java, with CompletableFuture and reactive programming models, offers powerful capabilities but with a steeper learning curve due to its verbose syntax.
- Performance & Scalability: Python and Node.js both offer high performance for I/O-bound applications due to their non-blocking nature. Go excels in performance and scalability due to its lightweight goroutines and efficient CPU utilization, making it suitable for high-load systems. Java, particularly with reactive frameworks, provides high performance and scalability, though at the cost of complexity.
- Ecosystem: Node.js boasts a very rich ecosystem with a vast number of libraries for almost any need, due to its popularity and the npm package manager. Python also has a rich ecosystem, especially for web development and data processing. Go's standard library is powerful, and its ecosystem is growing, especially in the cloud infrastructure space. Java's ecosystem is mature and extensive, with a wide range of libraries and frameworks for enterprise-level applications.
- Community Support: All four technologies have strong community support, with Node.js and Java being particularly notable due to their long-standing presence in the industry. Python's community is vibrant, especially in web development, data science, and AI. Go's community is growing rapidly, especially among developers working on distributed systems and microservices.
- Ideal Use Case: Python is ideal for web servers and asynchronous data processing tasks, Node.js excels in real-time applications and SPAs (Single Page Applications), Go is preferred for building concurrent services and networked applications due to its efficient concurrency model, and Java is suited for traditional web applications and microservices, especially where an extensive ecosystem of tools and libraries is required.
This table provides a high-level comparison, but the best choice depends on specific project requirements, existing expertise, and the application's particular scalability, performance, and maintainability needs.Deciding between Python vs. .NET for your business? Dive into our comprehensive comparison to determine which coding language best aligns with your project goals and accelerates your success!
Conclusion
Adopting async/await in Python for building high-performance I/O applications offers many benefits, from improved performance and efficiency to better resource utilization. Python developers can leverage these powerful features to build scalable and responsive applications by understanding the basics of asynchronous programming and following best practices. As Python continues to evolve, the async/await syntax is a testament to its commitment to supporting modern software development needs.
Author
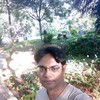