Apply ReactJS reusable component best practices of 2024, and enhance user experience, scalability and development efficiency.
Blog Overview: ReactJS reusable components are essential building blocks in modern front-end development, offering consistency, scalability, and efficiency across projects. In this blog, we delve into the best practices for styling and theming these components in 2024, exploring how adherence to these practices can streamline development workflows and enhance application maintainability.
ReactJS reusable components can be used across different application parts in projects. They exhibit similar traits, themes, styling, and behaviors, making them the ideal choice for reuse in various applications
For example, ReactJS buttons can use the same color, or forms can have the same input fields. With React, you can customize and use them in different projects without writing duplicate code.
There are many benefits to using ReactJS reusable components and React has gained a lot of popularity for designing user interfaces in the front-end development community. Today, we will explore some of the best practices for building ReactJS reusable components in 2024 and more below.
Benefits of Using Reusable ReactJS Components Best Practices
Here are the benefits of styling and theming ReactJS components in 2024 by leveraging the best practices:
1.Better User Experience
Using ReactJS reusable components and following the best practices for building them can ensure consistency throughout your codebase. Your applications will look more uniform and perform as expected. There are no unpleasant surprises to watch out for; it also helps reduce code redundancy. You can promote standardization across the entire project by ensuring identical design patterns, functionalities, and styles.
2.Improved Scalability
ReactJS reusable components' best practices make scaling and adapting applications to projects easier as they grow. It becomes easier to add new features and manage complex functionalities. Everyone on the team is on the same page, and it facilitates seamless collaboration between team members working on the same project.
3.Save Time and Money
Developers can save time and money by adopting ReactJS reusable components best practices 2024. They eliminate the need to rewrite code from scratch repeatedly. It's just a matter of writing once and reusing it multiple times. Organizations can accelerate project development and achieve milestones faster without compromising quality or performance.
Best Practices for Styling ReactJS Components
Following is a list of the best practices for styling ReactJS components and achieving code modularity.
1.CSS-in-JS Libraries
You can write different styles in JavaScript and apply them to ReactJS components. Some popular CSS-in-JS for ReactJS is emotion and styled-components. Styled components for ReactJS are automatically scoped as well.
2.Naming Conventions
You can use popular naming conventions to keep styles organized and easy to understand and ensure consistency in code. The best conventions are scalable and Modular Architecture for CSS (SMACSS) and Block-Element Modifier (BEM).
Use linters to check the code for styling and syntax errors. The best ones for developers are Stylelint, ESLint, and CSSLint.
3.Props for Customization
Props will help you customize the behavior and appearance of ReactJS reusable components. Creating components with a single responsibility and keeping them small and focused is an excellent practice.
Break down complex UI components into smaller, reusable sub-components and aim for modularity. It will ensure a clear and consistent hierarchy.
You can use props to modify data to change the UI rendering without changing any code. The easiest way to do that is by passing data through props, which also helps with code debugging.
Here is an example of how to pass data through props for a JSX button component.
4. Documentation
Creating good documentation is one of the top ReactJS reusable components best practices because it proves how your components interact and work. Other developers new to the project can contribute to the existing codebase, and live documentation can show what props various components accept. Good documentation will demonstrate how reusable components work in different scenarios and map their behaviors.
5. Testing
Testing is essential to building theming ReactJS components in 2024 and other UI elements. By testing your reusable components regularly, you can ensure they are bugs-free. It helps catch errors early on, and you may need to write individual tests for specific components.
When state changes or interactions occur, your tests should produce different results based on specific scenarios. It depends on the context, and many testing tools like Enzyme and Jest can automate the testing process.
6.Accessibility Guidelines
We recommend following the Web Content Accessibility Guidelines (WCAG 2) for building ReactJS reusable components. It is a good practice to use ARIA attributes and semantic HTML.
It's essential to ensure that ReactJS reusable components are accessible to all users, including populations with disabilities and hearing or speech impairments.
The WCAG 2 guidelines serve as a handy guide with testable criteria for each. It specifies where components and content meet the required specifications. It is also approved under the ISO standard and is a part of ReactJS reusable components best practices 2024. You can view the latest published version here.
Using React Hooks to Build Reusable UI Components
React v16.8 and later versions come with a new called Hooks. Hooks include functions that provide effective state management capabilities and work well with React’s lifecycle methods.
Different built-in Hooks are available, and developers can create custom hooks.
Hooks will allow functional components to perform side effects and help write reusable and stateful logic. The main types of Hooks are:
- State Hooks (useState)
- Context Hooks (useContext)
- Effect Hooks (useEffect)
- Performance Hooks (useMemo)
- Ref Hooks (useRef)
Below, we will cover a few ways to build reusable components using React Hooks.
1. Input Component
You can create reusable forms using the useForm hook from react-hook-form and import custom properties. Developers can pass input components to a prop and determine what inputs applications should render.
To get started, use this custom template to create a new project. Make a components folder with a FormInput.jsx file and paste the following code in it:
// ./components/FormInput.jsx
import { useState } from "react";
const inputStyle = {
padding: '8px',
border: '1px solid #ccc',
borderRadius: '4px',
color: '#333',
backgroundColor: '#fff',
width: '250px',
display: 'block',
};
function FormInput(props) {
const [inputType] = useState(props.type);
const [inputValue, setInputValue] = useState('');
function handleChange(event) {
const newValue = event.target.value
setInputValue(newValue);
if (props.onChange) props.onChange(newValue);
};
return (
<>
<span>{props?.title? props.title : "Untitled input"}: </span>
<input
type={inputType}
value={inputValue}
name="input-form"
onChange={handleChange}
placeholder={props?.placeholder}
autoComplete={props?.autocomplete ? props.autocomplete : "off"}
style={inputStyle} />
<br />
</>
);
};
2. Custom select component
To make a custom select component, create a CustomSelect.jsx file and save it in your components folder. Just paste the following code now:
// ./components/CustomSelect.jsx
import { useState } from "react";
const selectStyle = {
padding: '8px',
border: '1px solid #ccc',
borderRadius: '4px',
fontSize: '16px',
color: '#333',
backgroundColor: '#fff',
width: '250px',
display:'block',
};
function CustomSelect(props) {
const [data] = useState(props.data);
let options = data.map((item, index) => (
<option key={index} value={item}>{item}</option>
));
return (
<>
<select
name="customSearch"
onChange={(event) => props?.onSelectChange(event)}
style={selectStyle}>
<option value="">{props.title}</option>
{options}
</select>
<br />
</>
);
}
export default CustomSelect;
3.Button Component
You can make reusable button components using React Hooks and display different color variants and sizes for application use. Use the children property to display the contents of the button dynamically.
Create a Button.jsx file in your components folder and write the following code to it:
// ./components/Button.jsx
import { useState } from "react";
function Button(props) {
const [size] = useState(props.size);
const [variant] = useState(props.variant);
const buttonStyle = {
border: 'none',
borderRadius: '4px',
fontSize: '8px',
color: '#fff',
backgroundColor: '#54a0ff',
cursor: 'pointer'
};
if (props.size === 'lg') {
buttonStyle.height = "40px";
buttonStyle.fontSize = "18px";
}else if (props.size === 'sm') {
buttonStyle.height = "16px";
buttonStyle.fontSize = "10px";
}
if (props?.variant === "warning") buttonStyle.backgroundColor = "#ff0000"
if (props?.variant === "success") buttonStyle.backgroundColor = "#2ecc71"
return (
<button type={props.type} onClick={props?.onClick} style={buttonStyle}>
{props.children}
</button>
);
}
export default Button;
Combining Multiple Reusable Components
You can stitch together multiple reusable components using FormWrapper as your parent component. Any reusable component you put inside it will be its child.
To do this, create a file outside the App.jsx components folder and add the following code to it:
// ./App.jsx
import { useState } from "react";
import FormInput from "./components/FormInput.jsx";
import CustomSelect from "./components/CustomSelect.jsx";
import Button from "./components/Button.jsx";
import FormWrapper from "./components/FormWrapper.jsx";
import SearchBar from "./components/SearchBar.jsx";
import Modal from "./components/Modal.jsx";
import ToggleSwitch from './components/ToggleSwitch.jsx';
function App() {
const [formTwoSearch, modify_formTwoSearch] = useState("");
const [isModalShowing, setIsModalShowing] = useState(false);
const data = [ "One", "Two", "Three", "Four" ];
const handleChange = (value) => console.log(value);
const onToggleChange = (status) => console.log({ toggle: status });
const formOneSubmitter = ({ event }) => {
console.log("Form One can be submitted");
event.preventDefault();
};
const formTwoSubmitter = ({ event }) => {
console.log("Form Two can be submitted");
event.preventDefault();
};
return (
<>
<FormWrapper onSubmit={formOneSubmitter} title="Form One">
<CustomSelect
data={data}
title="Select number of bedrooms"
onSelectChange={(event) => console.log(`Got ${event.target.value}`)}
/>
<FormInput title="First Name" type={"text"} onChange={handleChange} autocomplete={"given-name"} />
<FormInput title="Last Name" type={"text"} onChange={handleChange} autocomplete={"family-name"} />
<FormInput title="Email address" type={"email"} onChange={handleChange} autocomplete={false} />
<FormInput title="Enter password" type={"password"} onChange={handleChange} />
<ToggleSwitch disabled={false} defaultChecked={true} onToggleChange={onToggleChange} OnTitle="Agree" OffTitle="Disagree" />
<Button type="submit" variant="primary" size={"lg"}> Submit data </Button>
</FormWrapper>
<FormWrapper onSubmit={formTwoSubmitter} title="Form Two">
<SearchBar
placeHolder="Find a house near me"
onSearch={(searchTerm) => modify_formTwoSearch(searchTerm)}
/>
<Button type="submit" variant="success" size={"sm"}> Search </Button>
<Button type="reset" variant="warning" size={"sm"} onClick={()=>modify_formTwoSearch("")}> Clear Form </Button>
{formTwoSearch && (
<p>
Searching for "<b>{formTwoSearch}</b>"
</p>
)}
</FormWrapper>
<>
<Button onClick={() => setIsModalShowing(true)}>View modal</Button>
<Modal isShowing={isModalShowing} onClose={() => setIsModalShowing(false)}>
<h3>About this app</h3>
<p> Try creating reusable components now!.</p>
<Button>
<a href="https://blog.logrocket.com/author/daggieblanqx/">
See more articles
</a>
</Button>
<Button onClick={() => setIsModalShowing(false)}>Close Modal</Button>
</Modal>
</>
</>
);
}
export default App;
Conclusion
Incorporating the best practices for styling ReactJS components in 2024 can accelerate your project development, maximize efficiency, and create maintainable applications. Following these practices will make components management simpler. It will help you build UI components that can be reused for various applications.
To hire ReactJS developers for your next project, contact Clarion Technologies.
Author
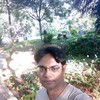