A REST API sets the rules for creating new web services and enables communication between different clients. REST is based on the architectural style for designing network-based software and stands for Representational State Transfer (REST) technology. One of the main advantages of making REST APIs in PHP is that they can be seamlessly integrated with other systems and technologies.
You can use simple HTTP requests to retrieve data from web servers and connect PHP apps with other services. REST APIs can be used with any programming language and are platform independent.
Rules for Creating REST APIs
REST APIS have certain rules that are to be followed which are known as design constraints. These constraints are parameters that define how these APIs are built, used, and deployed. The following are the key constraints that apply to REST APIs:
1. Uniform Interface: All resources used with REST APIs are connected and accessed using the same methods. REST APIs feature consistent interfaces, regardless of the underlying technologies that are used with them.
2. Client-Server: Client-Server components in REST APIs are separate and are developed independently. One component can be replaced with the other and doesn’t affect function or performance.
3. Stateless: Clients that issue API requests store all the necessary information and web servers do not store client contexts between requests. The stateless constraint makes it possible to scale existing web servers and add new web servers without needing to maintain client states.
4. Cacheable: The cacheable constraint is used to reduce server loads and enhance API performance, and cache API responses. It includes information about how the API is to be cached and for how long.
5. Code on Demand: The Code on Demand constraint gives developers the ability to download code to be executed on the client. It offers more advanced functionalities with the API without needing help from the client.
6. Layered System: The layered system constraint that APIs are meant to be designed using a layered approach. Each layer adds additional functionality and developers can add or remove new or existing functionalities as needed.
Factors to Consider Before Building a REST API
Before building a REST API with PHP, it’s important to carefully select a suitable framework. The framework should have the features needed to build, test, and maintain REST APIs. It should also be relatively straightforward to use and easy to implement for developers of all skill levels. Consider the version of PHP you are using and check to see if the framework you select is compatible with it before deciding to build REST APIs with it.
Finally, the framework should provide active community support and have plenty of resources, documentation, tutorials, and user support. It needs to be well-maintained and you can assess whether the framework is reliable based on the number of bug fixes and how quickly security issues get addressed by its creators.
The top PHP frameworks we recommend for creating REST APIs are Laravel, Slim, and Lumen.
How to Build a REST API
You can build a REST API in PHP using just a few lines of code. Here is how to get started:
1. Download Python (if you haven’t already) and install it on your computer. The first step is to set up a web server using XAMPP which you can download here. Install it after downloading.
2. Run XAMPP and boot up the Apache Web Server:
3. Navigate to the htdocs directory and create a folder inside it called rest-api. Now open it using your preferred code editor.
4. Make a directory called server within rest-api and create another directory called api inside it. Inside the api directory, create a hello.php file. This is where you will define the routes. Copy and paste this code:
In the code snippet above, you can see that the access control origin header has been set to all. You can check the REQUEST method to determine which HTTP method will request. Now anyone can call the API and once this is done, you can go to this URL.
You should get the following API response:
The GET request will execute when you call the API via the browser’s address bar. This is because you have made a GET request directly to the API.
Congratulations! With this, you have now created a simple REST API in PHP. You can start creating your own PHP-based REST APIs from here on out.
How Does the REST API Work?
REST requests are connected to CRUD operations and involve using the GET, PUT, POST, and DELETE requests. You can use GET to retrieve information, which is similar to Read, POST is used to make new records the same as Create and PUT is used to update records just like Update. The DELE TE request is used to delete records the same as Delete.
JSON file format is the most common output for the REST API.
How to Create a REST API in PHP with a Database and Table
If you want to create a PHP-based REST API with a database and table, you can do so by taking the following steps:
1. Create a database with dummy data by running the following query:
2. Create a table using the following code:
3. Build a database connection and create a db.php file. You can use the following code for this and update it using your latest database credentials:
4. Create an api.php file and paste the following script:
5. Once you have pasted the above script, you will be able to use the GET request on your API and return output in JSON format. You can create a folder called REST and view transaction data by visiting the following URL:
http://localhost/rest/api.php?order_id=15478959
6. You should get this output:
You can rewrite the above URL using the .htaccess file since it’s not user-friendly. Just copy paste the following code:
You can now view the REST API transaction data by visiting the following URL:
http://localhost/rest/api/15478959
Your output will now look like this which means your REST API is ready to use:
You can use this REST API to access and connect with web-based services. Keep in mind that REST APIs are not secure by default, and you will need to implement additional security measures to mitigate SQL injection attacks and other security issues.
These are two ways you can create simple REST APIs with PHP.
Where Can You Use REST APIs?
You can integrate REST APIs on social media platforms like Twitter and Facebook to view and access user data, statuses, lists, and more. In the e-Commerce business, REST APIs can be integrated with popular platforms like Shopify and Magento to view, access, and manage product catalogues and customer data. PHP-based REST APIs work well with content management systems like Drupal and WordPress and allows users to view blog posts, web pages, and other informational content on websites. Salesforce and HubSpot are customer relationship management systems that use REST APIs and REST APIs can also be used to power mobile apps.
Conclusion
As you can see, creating a REST API in PHP takes minutes and it is easy to get started. REST APIs can greatly personalize customer experiences and make it convenient to store and share information in secure, seamless, and accessible ways. They are an excellent choice for data access management and can display data across multiple platforms, thus making them highly versatile. The code snippets above should help you get started.
If you would like more PHP coding tips, tutorials, or hire PHP developers to get professional assistance with your upcoming projects, you can contact us. We help you build the best offshore technology teams in no time and provide various PHP development services such as PHP migration, custom software development, client onboarding services, and more. Our hiring model is very flexible, and we understand that every project has different requirements.
Author
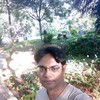